Kotlin for Seniors: 3 Powerful Features You Should Know! 🚀
Kotlin is packed with powerful features that make development smooth, expressive, and concise. Today, let’s dive into three cool Kotlin features that can make your code more elegant and readable: DSLs with infix functions, the invoke
operator, and delegation with by
.
Let's go! 🏎️
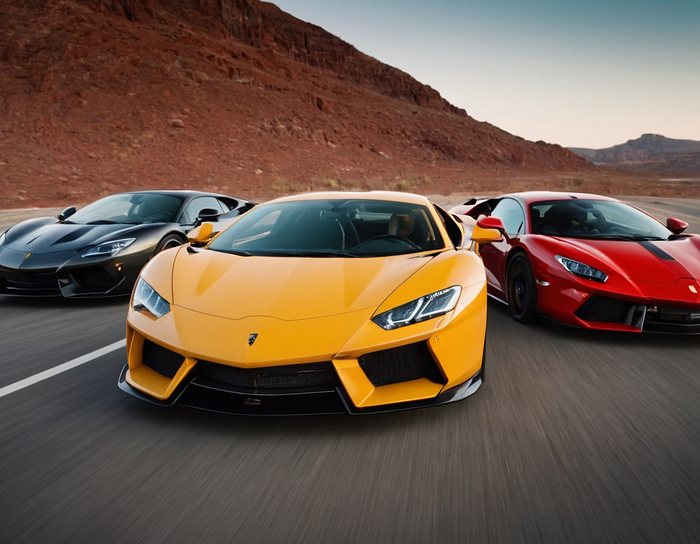
Domain-Specific Languages (DSLs) with Infix Functions 📜
One of Kotlin’s most powerful capabilities is creating DSLs (Domain-Specific Languages). DSLs allow you to write more readable, declarative code that feels natural. Kotlin’s infix functions make DSLs even cleaner!
class HtmlBuilder {
private val content = StringBuilder()
infix fun add(text: String) {
content.append(text).append("\n")
}
override fun toString(): String = content.toString()
}
fun html(init: HtmlBuilder.() -> Unit): HtmlBuilder {
val builder = HtmlBuilder()
builder.init()
return builder
}
fun main() {
val myHtml = html {
add "<h1>Hello, Kotlin DSL!</h1>"
add "<p>This is a paragraph.</p>"
}
println(myHtml)
}
👆 Here, the add
function is infix, making the DSL look clean and readable.
The invoke
Operator 🔥
In Kotlin, functions are first-class citizens, but did you know you can make objects callable like functions? Enter the invoke
operator!
class Greeter(val greeting: String) {
operator fun invoke(name: String) = "$greeting, $name!"
}
fun main() {
val sayHello = Greeter("Hello")
println(sayHello("Kotlin")) // Outputs: Hello, Kotlin!
}
This trick is especially useful in DSLs and higher-order functions. It lets you call instances as if they were functions!
Delegation with by
🔄
Delegation propeties:
import kotlin.properties.Delegates
class User {
var name: String by Delegates.observable("Unknown") { _, old, new ->
println("Name changed from $old to $new")
}
}
fun main() {
val user = User()
user.name = "Artem" // Prints: Name changed from Unknown to Artem
user.name = "Alex" // Prints: Name changed from Artem to Alex
}
Class delegation
interface Logger {
fun log(message: String)
}
class ConsoleLogger : Logger {
override fun log(message: String) = println("Logging: $message")
}
class App(logger: Logger) : Logger by logger
fun main() {
val app = App(ConsoleLogger())
app.log("Hello, Delegation!")
}
💡 Here, App
doesn’t need to implement log()
—it delegates it to ConsoleLogger
!
Wrapping Up 🎁
Kotlin is full of expressive and powerful features that can make your code more concise and elegant.
Which feature is your favorite? Let me know in the comments! 👇
💌folow me for more :)